Marigold Mobile SDK + Flutter
Integrate the Marigold Mobile SDK with a Flutter project.
The Marigold Mobile SDK is a natively built library for iOS and Android. Integrating this with a Flutter project consists of three parts.
- Add the native SDK to your project.
- Initialize the SDK connection with your SDK key (provided by Marigold) at the native code level
- Include and reference the example SDK Flutter project.
Add the native SDK to your project
Follow the steps outlined in the documentation for “Adding the Marigold Mobile SDK”.
https://sdkdevelopers.meetmarigold.com/docs/sdk-integration
There are separate guides for Android or iOS depending on which platforms you are building this for.
Android:
Include the necessary configuration elements in your build.gradle files.
See the Android Integration documentation for more information.
iOS:
There are a few different ways in which the SDK can be included depending on how your
project is setup. See the iOS Integration documentation for more information.
Initialize the SDK in the native code
Example native code integrations are shown in our Flutter reference project:
https://github.com/sailthru/marigold-flutter-sdk-example
Android
example/android/app/src/main/java/com/marigold/sdk/flutterexample/ExampleApplication.kt
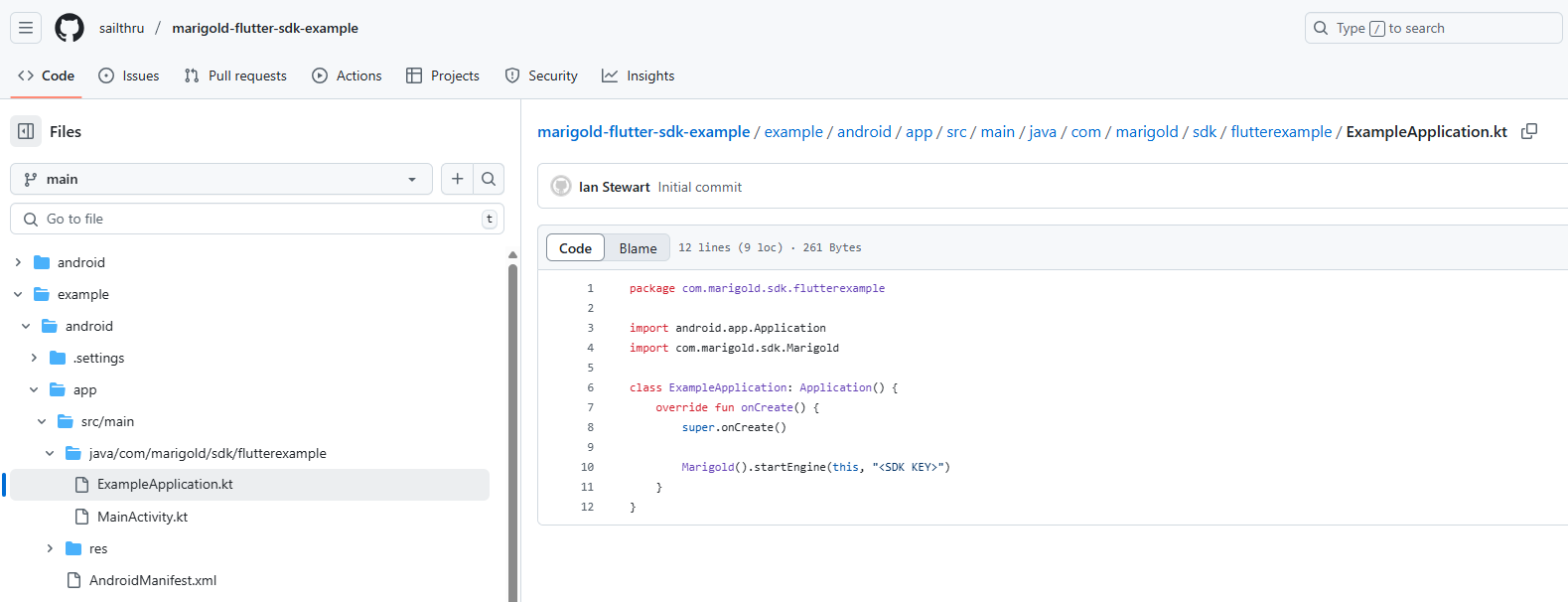
iOS
example/ios/Runner/AppDelegate.m
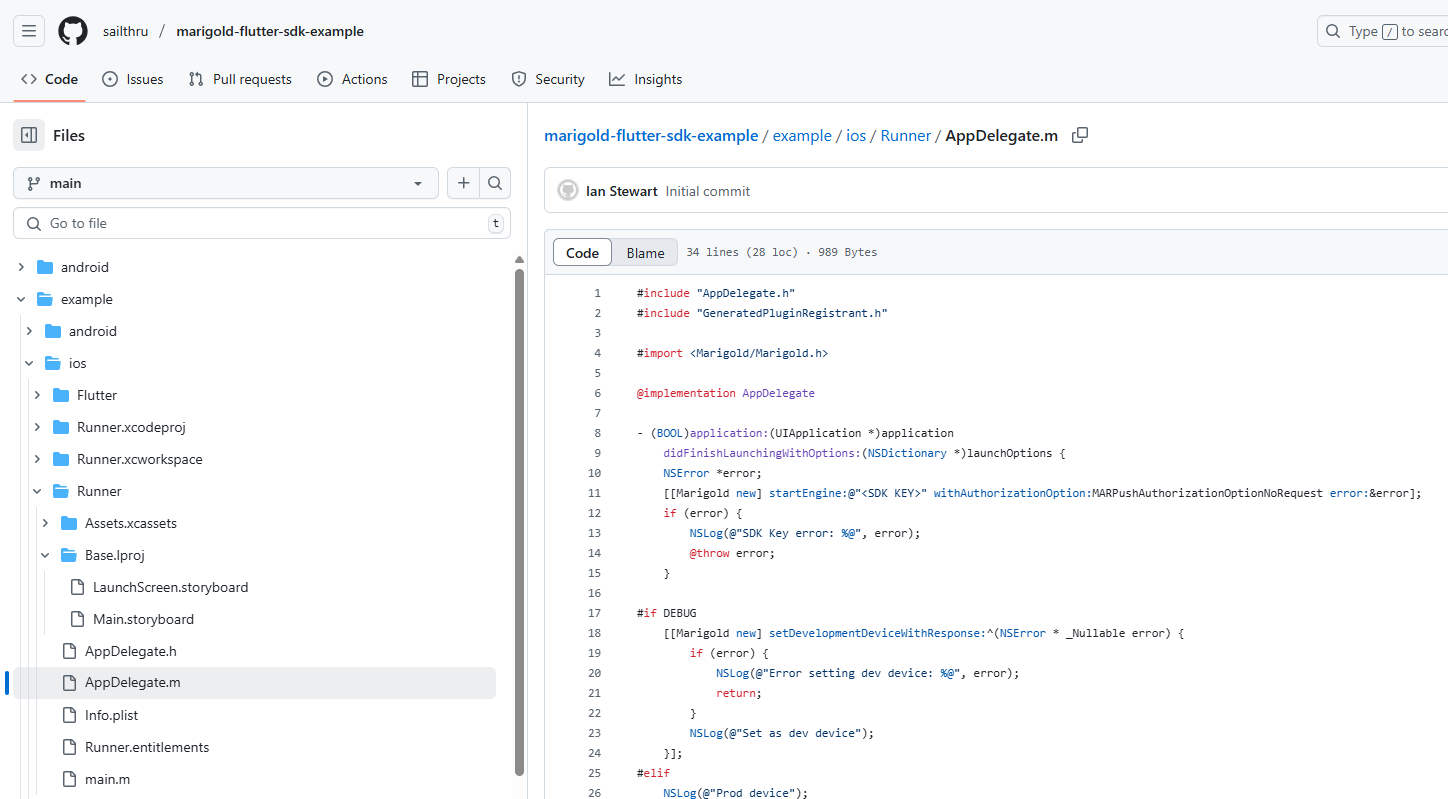
Integrate the example Flutter SDK project
The most straightforward way to integrate this into your project is to pull down the git repo to the root of your project folder.
The example below shows the repo being cloned on the command line of VS Studio to the root of the project named “demo1”
PS C:\projects\demo1> git clone https://github.com/sailthru/marigold-flutter-sdk-example.git marigold-flutter-sdk
Cloning into 'marigold-flutter-sdk'...
remote: Enumerating objects: 165, done.
remote: Counting objects: 100% (165/165), done.
remote: Compressing objects: 100% (113/113), done.
remote: Total 165 (delta 20), reused 165 (delta 20), pack-reused 0 (from 0)
Receiving objects: 100% (165/165), 130.72 KiB | 1.87 MiB/s, done.
Resolving deltas: 100% (20/20), done.
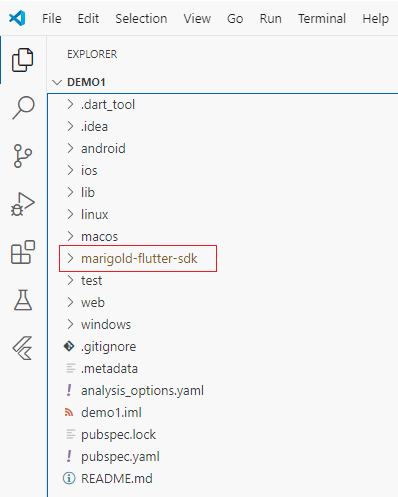
Add marigold_flutter_sdk
path to your project’s pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
marigold_flutter_sdk:
path: marigold-flutter-sdk/
Your project should now be able to reference the Marigold Mobile SDK using the flutter package reference “marigold_flutter_sdk”.
The sample code below shows how to call the logRegistrationEvent
referenced in the SDK documentation under collecting user data.
import 'package:marigold_flutter_sdk/marigold.dart';
void setUserId(String userId) async {
try {
await Marigold.logRegistrationEvent(userId);
} on Exception catch (e) {
print(e.toString());
}
}
Additional code examples are provided in the example project main.dart file.
Updated about 1 month ago